In this tutorial, you use Python 3 to create the simplest Python 'Hello World' application in Visual Studio Code. By using the Python extension, you make VS Code into a great lightweight Python IDE (which you may find a productive alternative to PyCharm).
Here are the steps to install XCode, HomeBrew as well as install Python 3 using HomeBrew onto your Mac: Jump into your Terminal app on your Mac and run the copy/paste the following command into the Terminal to install XCode onto your Mac: $ xcode-select -install; Simply click through all the confirmation crap that XCode shows. Kivy is an open-source Python library; you can use it to create applications on Windows, Linux, macOS, Android, and iOS. We will discuss how to play with the Kivy buttons, labels, recycle view, scroll view, Kivy Canvas, and other widgets to become familiar with the library. I need to be able to open a document using its default application in Windows and Mac OS. Basically, I want to do the same thing that happens when you double-click on the document icon in Explorer. 'open', filename) In Python 3.5+ you can equivalently use the slightly more complex but also somewhat more versatile.
This tutorial introduces you to VS Code as a Python environment, primarily how to edit, run, and debug code through the following tasks:
- Write, run, and debug a Python 'Hello World' Application
- Learn how to install packages by creating Python virtual environments
- Write a simple Python script to plot figures within VS Code
This tutorial is not intended to teach you Python itself. Once you are familiar with the basics of VS Code, you can then follow any of the programming tutorials on python.org within the context of VS Code for an introduction to the language.
If you have any problems, feel free to file an issue for this tutorial in the VS Code documentation repository.

Prerequisites
To successfully complete this tutorial, you need to first setup your Python development environment. Specifically, this tutorial requires:
- VS Code
- VS Code Python extension
- Python 3
Install Visual Studio Code and the Python Extension
If you have not already done so, install VS Code.
Next, install the Python extension for VS Code from the Visual Studio Marketplace. For additional details on installing extensions, see Extension Marketplace. The Python extension is named Python and it's published by Microsoft.
Install a Python interpreter
Run Python On Mac
Along with the Python extension, you need to install a Python interpreter. Which interpreter you use is dependent on your specific needs, but some guidance is provided below.
Windows
Install Python from python.org. You can typically use the Download Python button that appears first on the page to download the latest version.
Note: If you don't have admin access, an additional option for installing Python on Windows is to use the Microsoft Store. The Microsoft Store provides installs of Python 3.7 and Python 3.8. Be aware that you might have compatibility issues with some packages using this method.
For additional information about using Python on Windows, see Using Python on Windows at Python.org
macOS
The system install of Python on macOS is not supported. Instead, an installation through Homebrew is recommended. To install Python using Homebrew on macOS use brew install python3
at the Terminal prompt.
Note On macOS, make sure the location of your VS Code installation is included in your PATH environment variable. See these setup instructions for more information.
Linux
The built-in Python 3 installation on Linux works well, but to install other Python packages you must install pip
with get-pip.py.
Other options
Data Science: If your primary purpose for using Python is Data Science, then you might consider a download from Anaconda. Anaconda provides not just a Python interpreter, but many useful libraries and tools for data science.
Windows Subsystem for Linux: If you are working on Windows and want a Linux environment for working with Python, the Windows Subsystem for Linux (WSL) is an option for you. If you choose this option, you'll also want to install the Remote - WSL extension. For more information about using WSL with VS Code, see VS Code Remote Development or try the Working in WSL tutorial, which will walk you through setting up WSL, installing Python, and creating a Hello World application running in WSL.
Verify the Python installation
To verify that you've installed Python successfully on your machine, run one of the following commands (depending on your operating system):
Linux/macOS: open a Terminal Window and type the following command:
Windows: open a command prompt and run the following command:
If the installation was successful, the output window should show the version of Python that you installed.
Note You can use the py -0
command in the VS Code integrated terminal to view the versions of python installed on your machine. The default interpreter is identified by an asterisk (*).
Start VS Code in a project (workspace) folder
Using a command prompt or terminal, create an empty folder called 'hello', navigate into it, and open VS Code (code
) in that folder (.
) by entering the following commands:
Note: If you're using an Anaconda distribution, be sure to use an Anaconda command prompt.
By starting VS Code in a folder, that folder becomes your 'workspace'. VS Code stores settings that are specific to that workspace in .vscode/settings.json
, which are separate from user settings that are stored globally.
Alternately, you can run VS Code through the operating system UI, then use File > Open Folder to open the project folder.
Select a Python interpreter
Python is an interpreted language, and in order to run Python code and get Python IntelliSense, you must tell VS Code which interpreter to use.
From within VS Code, select a Python 3 interpreter by opening the Command Palette (⇧⌘P (Windows, Linux Ctrl+Shift+P)), start typing the Python: Select Interpreter command to search, then select the command. You can also use the Select Python Environment option on the Status Bar if available (it may already show a selected interpreter, too):
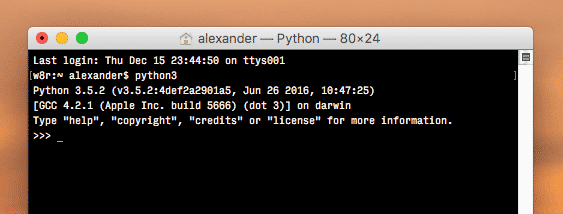
The command presents a list of available interpreters that VS Code can find automatically, including virtual environments. If you don't see the desired interpreter, see Configuring Python environments.
Note: When using an Anaconda distribution, the correct interpreter should have the suffix ('base':conda)
, for example Python 3.7.3 64-bit ('base':conda)
.
Selecting an interpreter sets the python.pythonPath
value in your workspace settings to the path of the interpreter. To see the setting, select File > Preferences > Settings (Code > Preferences > Settings on macOS), then select the Workspace Settings tab.
Note: If you select an interpreter without a workspace folder open, VS Code sets python.pythonPath
in your user settings instead, which sets the default interpreter for VS Code in general. The user setting makes sure you always have a default interpreter for Python projects. The workspace settings lets you override the user setting.
Create a Python Hello World source code file
From the File Explorer toolbar, select the New File button on the hello
folder:
Name the file hello.py
, and it automatically opens in the editor:
By using the .py
file extension, you tell VS Code to interpret this file as a Python program, so that it evaluates the contents with the Python extension and the selected interpreter.
Note: The File Explorer toolbar also allows you to create folders within your workspace to better organize your code. You can use the New folder button to quickly create a folder.
Now that you have a code file in your Workspace, enter the following source code in hello.py
:
When you start typing print
, notice how IntelliSense presents auto-completion options.
IntelliSense and auto-completions work for standard Python modules as well as other packages you've installed into the environment of the selected Python interpreter. It also provides completions for methods available on object types. For example, because the msg
variable contains a string, IntelliSense provides string methods when you type msg.
:
Feel free to experiment with IntelliSense some more, but then revert your changes so you have only the msg
variable and the print
call, and save the file (⌘S (Windows, Linux Ctrl+S)).
For full details on editing, formatting, and refactoring, see Editing code. The Python extension also has full support for Linting.
Run Hello World
It's simple to run hello.py
with Python. Just click the Run Python File in Terminal play button in the top-right side of the editor.
The button opens a terminal panel in which your Python interpreter is automatically activated, then runs python3 hello.py
(macOS/Linux) or python hello.py
(Windows):
There are three other ways you can run Python code within VS Code:
Right-click anywhere in the editor window and select Run Python File in Terminal (which saves the file automatically):
Select one or more lines, then press Shift+Enter or right-click and select Run Selection/Line in Python Terminal. This command is convenient for testing just a part of a file.
From the Command Palette (⇧⌘P (Windows, Linux Ctrl+Shift+P)), select the Python: Start REPL command to open a REPL terminal for the currently selected Python interpreter. In the REPL, you can then enter and run lines of code one at a time.
Configure and run the debugger
Let's now try debugging our simple Hello World program.
First, set a breakpoint on line 2 of hello.py
by placing the cursor on the print
call and pressing F9. Alternately, just click in the editor's left gutter, next to the line numbers. When you set a breakpoint, a red circle appears in the gutter.
Next, to initialize the debugger, press F5. Since this is your first time debugging this file, a configuration menu will open from the Command Palette allowing you to select the type of debug configuration you would like for the opened file.
Note: VS Code uses JSON files for all of its various configurations; launch.json
is the standard name for a file containing debugging configurations.
These different configurations are fully explained in Debugging configurations; for now, just select Python File, which is the configuration that runs the current file shown in the editor using the currently selected Python interpreter.
The debugger will stop at the first line of the file breakpoint. The current line is indicated with a yellow arrow in the left margin. If you examine the Local variables window at this point, you will see now defined msg
variable appears in the Local pane.
A debug toolbar appears along the top with the following commands from left to right: continue (F5), step over (F10), step into (F11), step out (⇧F11 (Windows, Linux Shift+F11)), restart (⇧⌘F5 (Windows, Linux Ctrl+Shift+F5)), and stop (⇧F5 (Windows, Linux Shift+F5)).
The Status Bar also changes color (orange in many themes) to indicate that you're in debug mode. The Python Debug Console also appears automatically in the lower right panel to show the commands being run, along with the program output.
To continue running the program, select the continue command on the debug toolbar (F5). The debugger runs the program to the end.
Tip Debugging information can also be seen by hovering over code, such as variables. In the case of msg
, hovering over the variable will display the string Hello world
in a box above the variable.
You can also work with variables in the Debug Console (If you don't see it, select Debug Console in the lower right area of VS Code, or select it from the ... menu.) Then try entering the following lines, one by one, at the > prompt at the bottom of the console:
Select the blue Continue button on the toolbar again (or press F5) to run the program to completion. 'Hello World' appears in the Python Debug Console if you switch back to it, and VS Code exits debugging mode once the program is complete.
If you restart the debugger, the debugger again stops on the first breakpoint.
To stop running a program before it's complete, use the red square stop button on the debug toolbar (⇧F5 (Windows, Linux Shift+F5)), or use the Run > Stop debugging menu command.
For full details, see Debugging configurations, which includes notes on how to use a specific Python interpreter for debugging.
Tip: Use Logpoints instead of print statements: Developers often litter source code with print
statements to quickly inspect variables without necessarily stepping through each line of code in a debugger. In VS Code, you can instead use Logpoints. A Logpoint is like a breakpoint except that it logs a message to the console and doesn't stop the program. For more information, see Logpoints in the main VS Code debugging article.
Install and use packages
Let's now run an example that's a little more interesting. In Python, packages are how you obtain any number of useful code libraries, typically from PyPI. For this example, you use the matplotlib
and numpy
packages to create a graphical plot as is commonly done with data science. (Note that matplotlib
cannot show graphs when running in the Windows Subsystem for Linux as it lacks the necessary UI support.)
Return to the Explorer view (the top-most icon on the left side, which shows files), create a new file called standardplot.py
, and paste in the following source code:
Tip: If you enter the above code by hand, you may find that auto-completions change the names after the as
keywords when you press Enter at the end of a line. To avoid this, type a space, then Enter.
Next, try running the file in the debugger using the 'Python: Current file' configuration as described in the last section.
Unless you're using an Anaconda distribution or have previously installed the matplotlib
package, you should see the message, 'ModuleNotFoundError: No module named 'matplotlib'. Such a message indicates that the required package isn't available in your system.
To install the matplotlib
package (which also installs numpy
as a dependency), stop the debugger and use the Command Palette to run Terminal: Create New Integrated Terminal (⌃⇧` (Windows, Linux Ctrl+Shift+`)). This command opens a command prompt for your selected interpreter.
A best practice among Python developers is to avoid installing packages into a global interpreter environment. You instead use a project-specific virtual environment
that contains a copy of a global interpreter. Once you activate that environment, any packages you then install are isolated from other environments. Such isolation reduces many complications that can arise from conflicting package versions. To create a virtual environment and install the required packages, enter the following commands as appropriate for your operating system:
Note: For additional information about virtual environments, see Environments.
Create and activate the virtual environment
Note: When you create a new virtual environment, you should be prompted by VS Code to set it as the default for your workspace folder. If selected, the environment will automatically be activated when you open a new terminal.
For windows
If the activate command generates the message 'Activate.ps1 is not digitally signed. You cannot run this script on the current system.', then you need to temporarily change the PowerShell execution policy to allow scripts to run (see About Execution Policies in the PowerShell documentation):
For macOS/Linux
Select your new environment by using the Python: Select Interpreter command from the Command Palette.
Install the packages
Rerun the program now (with or without the debugger) and after a few moments a plot window appears with the output:
Once you are finished, type
deactivate
in the terminal window to deactivate the virtual environment.
For additional examples of creating and activating a virtual environment and installing packages, see the Django tutorial and the Flask tutorial.
Next steps
You can configure VS Code to use any Python environment you have installed, including virtual and conda environments. You can also use a separate environment for debugging. For full details, see Environments.
To learn more about the Python language, follow any of the programming tutorials listed on python.org within the context of VS Code.
To learn to build web apps with the Django and Flask frameworks, see the following tutorials:
There is then much more to explore with Python in Visual Studio Code:
- Editing code - Learn about autocomplete, IntelliSense, formatting, and refactoring for Python.
- Linting - Enable, configure, and apply a variety of Python linters.
- Debugging - Learn to debug Python both locally and remotely.
- Testing - Configure test environments and discover, run, and debug tests.
- Settings reference - Explore the full range of Python-related settings in VS Code.
The following is a step-by-step guide for beginners interested in learning Python using Windows 10.
Set up your development environment
For beginners who are new to Python, we recommend you install Python from the Microsoft Store. Installing via the Microsoft Store uses the basic Python3 interpreter, but handles set up of your PATH settings for the current user (avoiding the need for admin access), in addition to providing automatic updates. This is especially helpful if you are in an educational environment or a part of an organization that restricts permissions or administrative access on your machine.
If you are using Python on Windows for web development, we recommend a different set up for your development environment. Rather than installing directly on Windows, we recommend installing and using Python via the Windows Subsystem for Linux. For help, see: Get started using Python for web development on Windows. If you're interested in automating common tasks on your operating system, see our guide: Get started using Python on Windows for scripting and automation. For some advanced scenarios (like needing to access/modify Python's installed files, make copies of binaries, or use Python DLLs directly), you may want to consider downloading a specific Python release directly from python.org or consider installing an alternative, such as Anaconda, Jython, PyPy, WinPython, IronPython, etc. We only recommend this if you are a more advanced Python programmer with a specific reason for choosing an alternative implementation.
Install Python
To install Python using the Microsoft Store:
Go to your Start menu (lower left Windows icon), type 'Microsoft Store', select the link to open the store.
Once the store is open, select Search from the upper-right menu and enter 'Python'. Open 'Python 3.7' from the results under Apps. Select Get.
Once Python has completed the downloading and installation process, open Windows PowerShell using the Start menu (lower left Windows icon). Once PowerShell is open, enter
Python --version
to confirm that Python3 has installed on your machine.The Microsoft Store installation of Python includes pip, the standard package manager. Pip allows you to install and manage additional packages that are not part of the Python standard library. To confirm that you also have pip available to install and manage packages, enter
pip --version
.
Install Visual Studio Code
By using VS Code as your text editor / integrated development environment (IDE), you can take advantage of IntelliSense (a code completion aid), Linting (helps avoid making errors in your code), Debug support (helps you find errors in your code after you run it), Code snippets (templates for small reusable code blocks), and Unit testing (testing your code's interface with different types of input).
VS Code also contains a built-in terminal that enables you to open a Python command line with Windows Command prompt, PowerShell, or whatever you prefer, establishing a seamless workflow between your code editor and command line.
To install VS Code, download VS Code for Windows: https://code.visualstudio.com.
Once VS Code has been installed, you must also install the Python extension. To install the Python extension, you can select the VS Code Marketplace link or open VS Code and search for Python in the extensions menu (Ctrl+Shift+X).
Python is an interpreted language, and in order to run Python code, you must tell VS Code which interpreter to use. We recommend sticking with Python 3.7 unless you have a specific reason for choosing something different. Once you've installed the Python extension, select a Python 3 interpreter by opening the Command Palette (Ctrl+Shift+P), start typing the command Python: Select Interpreter to search, then select the command. You can also use the Select Python Environment option on the bottom Status Bar if available (it may already show a selected interpreter). The command presents a list of available interpreters that VS Code can find automatically, including virtual environments. If you don't see the desired interpreter, see Configuring Python environments.
To open the terminal in VS Code, select View > Terminal, or alternatively use the shortcut Ctrl+` (using the backtick character). The default terminal is PowerShell.
Inside your VS Code terminal, open Python by simply entering the command:
python
Try the Python interpreter out by entering:
print('Hello World')
. Python will return your statement 'Hello World'.
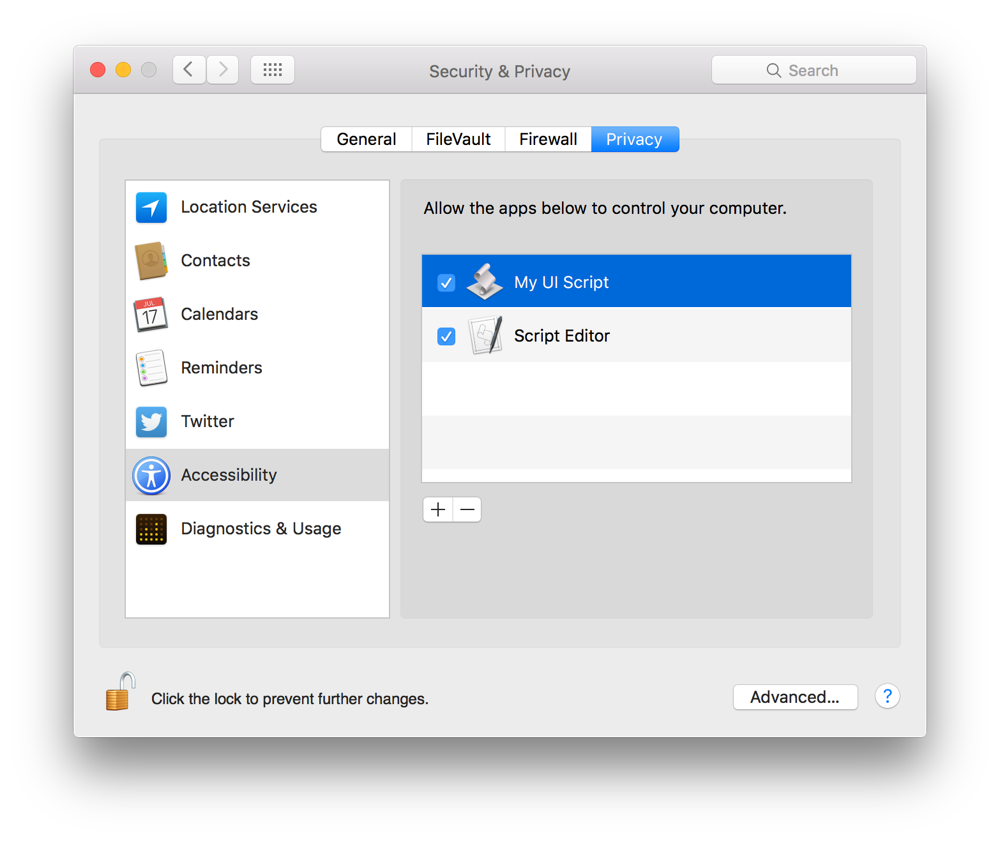
Install Git (optional)
If you plan to collaborate with others on your Python code, or host your project on an open-source site (like GitHub), VS Code supports version control with Git. The Source Control tab in VS Code tracks all of your changes and has common Git commands (add, commit, push, pull) built right into the UI. You first need to install Git to power the Source Control panel.
Download and install Git for Windows from the git-scm website.
An Install Wizard is included that will ask you a series of questions about settings for your Git installation. We recommend using all of the default settings, unless you have a specific reason for changing something.
If you've never worked with Git before, GitHub Guides can help you get started.
Hello World tutorial for some Python basics
Python, according to its creator Guido van Rossum, is a “high-level programming language, and its core design philosophy is all about code readability and a syntax which allows programmers to express concepts in a few lines of code.”
Python is an interpreted language. In contrast to compiled languages, in which the code you write needs to be translated into machine code in order to be run by your computer's processor, Python code is passed straight to an interpreter and run directly. You just type in your code and run it. Let's try it!
With your PowerShell command line open, enter
python
to run the Python 3 interpreter. (Some instructions prefer to use the commandpy
orpython3
, these should also work). You will know that you're successful because a >>> prompt with three greater-than symbols will display.There are several built-in methods that allow you to make modifications to strings in Python. Create a variable, with:
variable = 'Hello World!'
. Press Enter for a new line.Print your variable with:
print(variable)
. This will display the text 'Hello World!'.Find out the length, how many characters are used, of your string variable with:
len(variable)
. This will display that there are 12 characters used. (Note that the blank space it counted as a character in the total length.)Convert your string variable to upper-case letters:
variable.upper()
. Now convert your string variable to lower-case letters:variable.lower()
.Count how many times the letter 'l' is used in your string variable:
variable.count('l')
.Search for a specific character in your string variable, let's find the exclamation point, with:
variable.find('!')
. This will display that the exclamation point is found in the 11th position character of the string.Replace the exclamation point with a question mark:
variable.replace('!', '?')
.To exit Python, you can enter
exit()
,quit()
, or select Ctrl-Z.
Hope you had fun using some of Python's built-in string modification methods. Now try creating a Python program file and running it with VS Code.
Hello World tutorial for using Python with VS Code
The VS Code team has put together a great Getting Started with Python tutorial walking through how to create a Hello World program with Python, run the program file, configure and run the debugger, and install packages like matplotlib and numpy to create a graphical plot inside a virtual environment.
Open PowerShell and create an empty folder called 'hello', navigate into this folder, and open it in VS Code:
Once VS Code opens, displaying your new hello folder in the left-side Explorer window, open a command line window in the bottom panel of VS Code by pressing Ctrl+` (using the backtick character) or selecting View > Terminal. By starting VS Code in a folder, that folder becomes your 'workspace'. VS Code stores settings that are specific to that workspace in .vscode/settings.json, which are separate from user settings that are stored globally.
Continue the tutorial in the VS Code docs: Create a Python Hello World source code file.
Create a simple game with Pygame
Pygame is a popular Python package for writing games - encouraging students to learn programming while creating something fun. Pygame displays graphics in a new window, and so it will not work under the command-line-only approach of WSL. However, if you installed Python via the Microsoft Store as detailed in this tutorial, it will work fine.
Once you have Python installed, install pygame from the command line (or the terminal from within VS Code) by typing
python -m pip install -U pygame --user
.Test the installation by running a sample game :
python -m pygame.examples.aliens
All being well, the game will open a window. Close the window when you are done playing.
Download Python For Mac
Here's how to start writing your own game.
Open PowerShell (or Windows Command Prompt) and create an empty folder called 'bounce'. Navigate to this folder and create a file named 'bounce.py'. Open the folder in VS Code:
Using VS Code, enter the following Python code (or copy and paste it):
Save it as:
bounce.py
.From the PowerShell terminal, run it by entering:
python bounce.py
.
Try adjusting some of the numbers to see what effect they have on your bouncing ball.
Read more about writing games with pygame at pygame.org.
Resources for continued learning
We recommend the following resources to support you in continuing to learn about Python development on Windows.
Online courses for learning Python
Introduction to Python on Microsoft Learn: Try the interactive Microsoft Learn platform and earn experience points for completing this module covering the basics on how to write basic Python code, declare variables, and work with console input and output. The interactive sandbox environment makes this a great place to start for folks who don't have their Python development environment set up yet.
Python on Pluralsight: 8 Courses, 29 Hours: The Python learning path on Pluralsight offers online courses covering a variety of topics related to Python, including a tool to measure your skill and find your gaps.
LearnPython.org Tutorials: Get started on learning Python without needing to install or set anything up with these free interactive Python tutorials from the folks at DataCamp.
The Python.org Tutorials: Introduces the reader informally to the basic concepts and features of the Python language and system.
Learning Python on Lynda.com: A basic introduction to Python.
Python Mac Gui
Working with Python in VS Code
Download Python For Mac Os
Editing Python in VS Code: Learn more about how to take advantage of VS Code's autocomplete and IntelliSense support for Python, including how to customize their behavior... or just turn them off.
Linting Python: Linting is the process of running a program that will analyse code for potential errors. Learn about the different forms of linting support VS Code provides for Python and how to set it up.
Debugging Python: Debugging is the process of identifying and removing errors from a computer program. This article covers how to initialize and configure debugging for Python with VS Code, how to set and validate breakpoints, attach a local script, perform debugging for different app types or on a remote computer, and some basic troubleshooting.
Unit testing Python: Covers some background explaining what unit testing means, an example walkthrough, enabling a test framework, creating and running your tests, debugging tests, and test configuration settings.